In my first article I'll demonstrate how to create a project in Visual Studio for MS SharePoint server 2013 and make the buttons for authorization (Approve and Reject) in Ribbon for updating of list/library items.
In one of the projects I was asked to make it possible to authorize the list/library items when selecting these elements with the mouse in the list itself without opening one of the forms. Such a decision seemed to me interesting and I studied the CSOM with the possibility of a method SP.ListOperation.Selection.getSelectedItems().
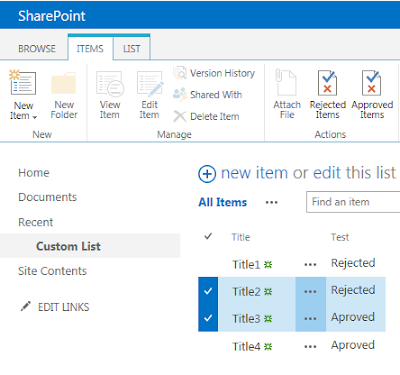
Further in the screenshots you can see that when you select multiple items and when you click button "Approved Items" or "Rejected Items", depending on the authorization logic the selected list items are updated.
Select "Title2" (Rejected) and "Title3" (Approved), click button "Approved Items, then will see: "Title3 is Approved"" and "Title2 successfully Approved".
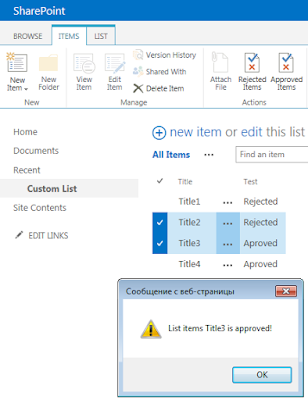
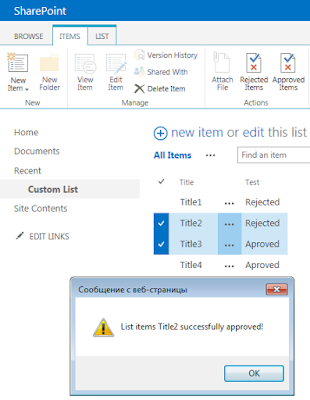
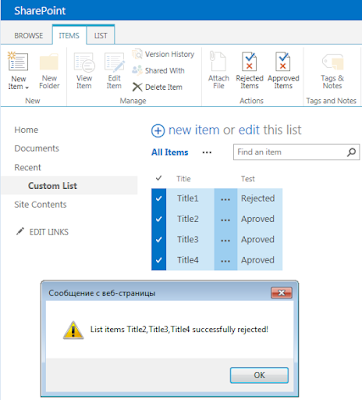
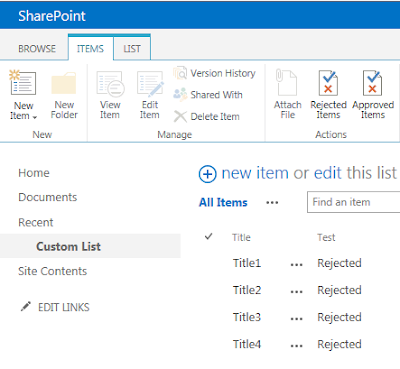
How is create of this solution, lets get started:
1. Open the visual studio 2012 or 2015 as run as administrator.
2. Create an Empty SharePoint Project 2013.
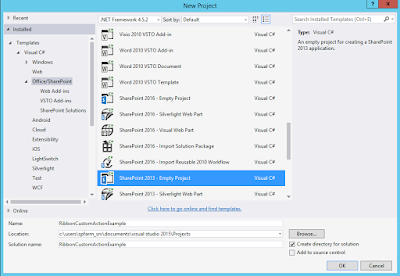
3. Let the name be "RibbonCustomActionExample".
4. Select a Farm Solution.
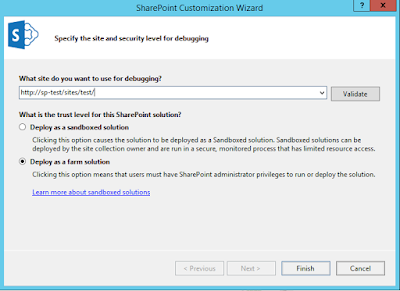
5. Add New Item to the solution (Module).
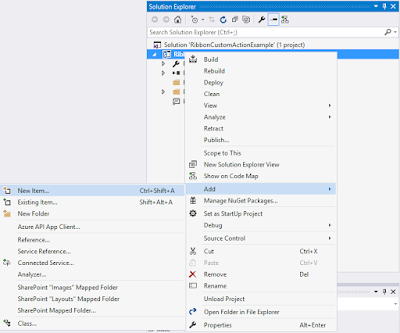
6. Add 2 Module. Name it as ApprovedCA
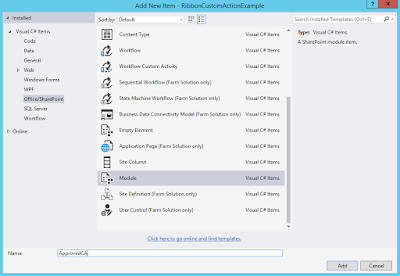
and RejectedCA
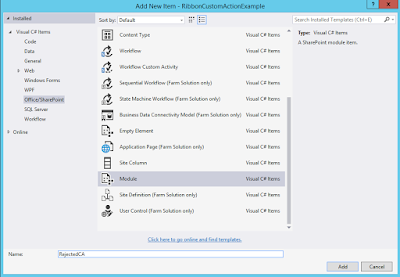
7. Then Add New Item to the solution (SharePoint "Layouts" Mapped Folder).
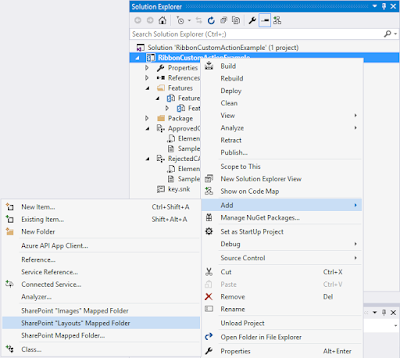
8. Rename folder "Layouts" in "Scripts".
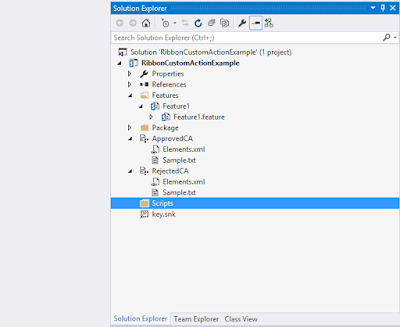
9. Add New Item to folder "Scripts".
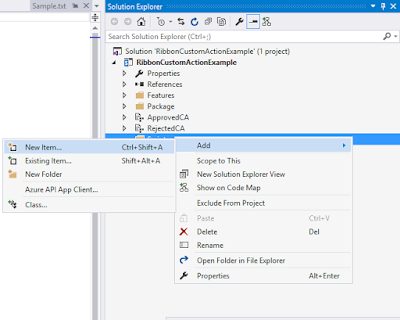
10. JavaScript-file name "script.js".
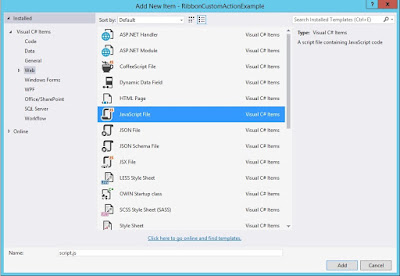
11. Open JavaScript-file "script.js" and add to code:
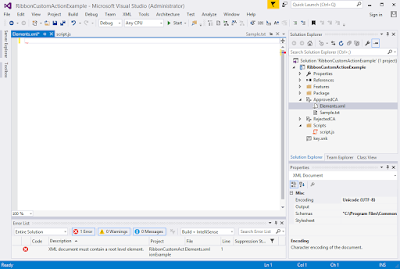
13. Add to code in this Elements.xml (ApprovedCA):
In one of the projects I was asked to make it possible to authorize the list/library items when selecting these elements with the mouse in the list itself without opening one of the forms. Such a decision seemed to me interesting and I studied the CSOM with the possibility of a method SP.ListOperation.Selection.getSelectedItems().
var context = SP.ClientContext.get_current();
var selectedItemIds = SP.ListOperation.Selection.getSelectedItems(context);
It allows you to work directly with the list items when you select a click.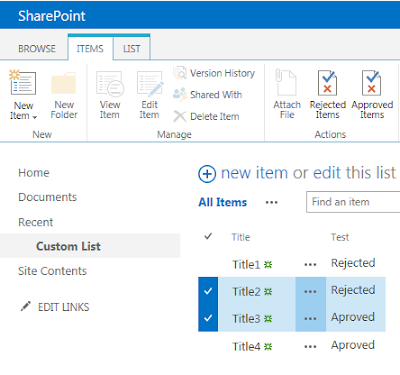
Further in the screenshots you can see that when you select multiple items and when you click button "Approved Items" or "Rejected Items", depending on the authorization logic the selected list items are updated.
Select "Title2" (Rejected) and "Title3" (Approved), click button "Approved Items, then will see: "Title3 is Approved"" and "Title2 successfully Approved".
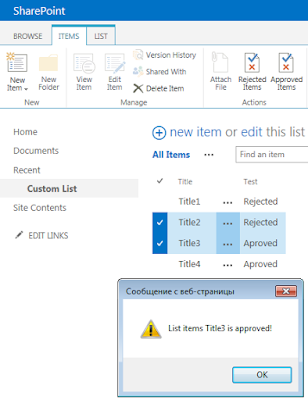
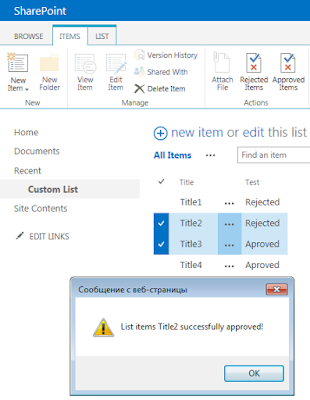
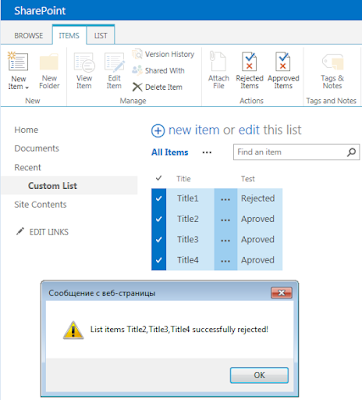
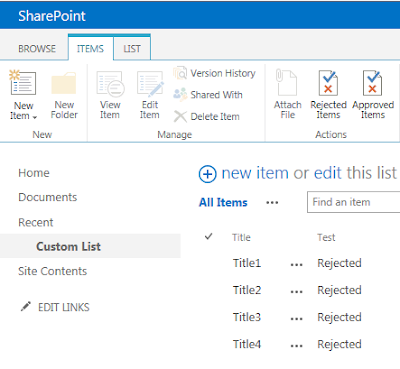
How is create of this solution, lets get started:
1. Open the visual studio 2012 or 2015 as run as administrator.
2. Create an Empty SharePoint Project 2013.
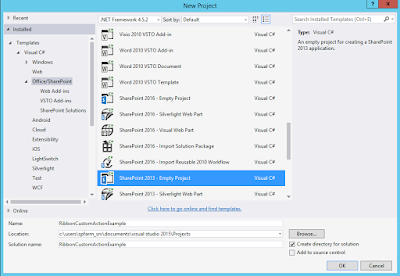
3. Let the name be "RibbonCustomActionExample".
4. Select a Farm Solution.
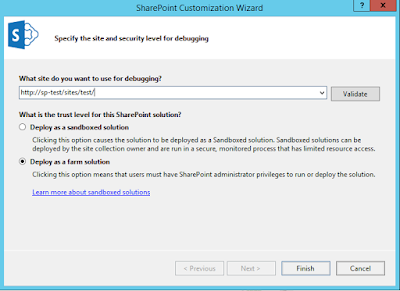
5. Add New Item to the solution (Module).
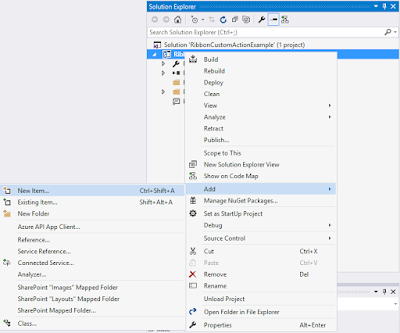
6. Add 2 Module. Name it as ApprovedCA
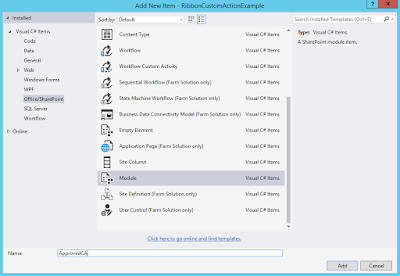
and RejectedCA
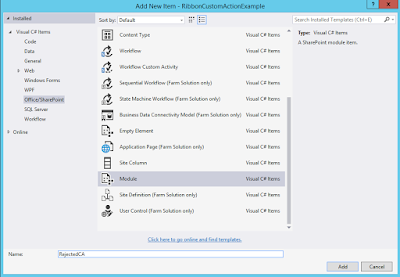
7. Then Add New Item to the solution (SharePoint "Layouts" Mapped Folder).
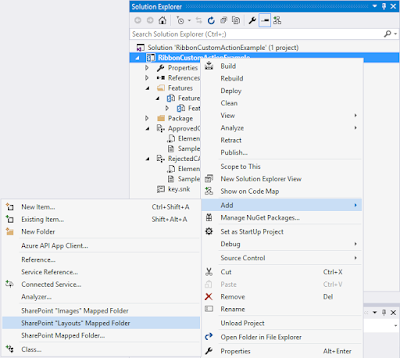
8. Rename folder "Layouts" in "Scripts".
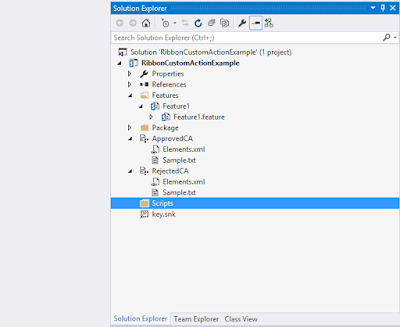
9. Add New Item to folder "Scripts".
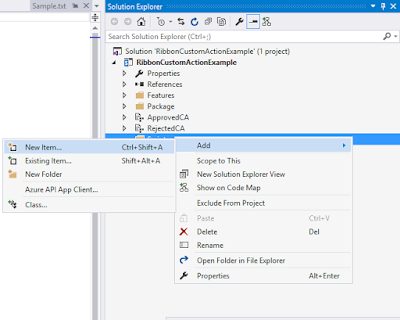
10. JavaScript-file name "script.js".
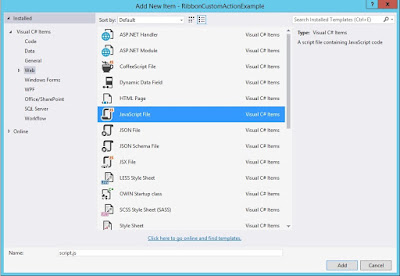
11. Open JavaScript-file "script.js" and add to code:
function getSelectedItems(OnSuccess, OnError) {
var context = SP.ClientContext.get_current();
var listId = SP.ListOperation.Selection.getSelectedList();
var selectedItemIds = SP.ListOperation.Selection.getSelectedItems(context);
var list = context.get_web().get_lists().getById(listId);
var listItems = [];
for (idx in selectedItemIds) {
var item = list.getItemById(parseInt(selectedItemIds[idx].id));
listItems.push(item);
context.load(item);
}
context.executeQueryAsync(
function () {
OnSuccess(listItems);
},
OnError
);
}
function RejectedItems() {
var context = SP.ClientContext.get_current();
var selectedItems = SP.ListOperation.Selection.getSelectedItems(context);
var ListGUID = SP.ListOperation.Selection.getSelectedList();
var clientContext = new SP.ClientContext.get_current();
var targetList = clientContext.get_web().get_lists().getById(ListGUID);
var itemArray = [];
var itemAllertNo = [];
var itemAllertYes = [];
getSelectedItems(function (items) {
for (var i = 0 ; i < items.length; i++) {
if ((items[i].get_item('Test') == "Rejected")) {
itemAllertNo.push(items[i].get_item('Title'));
} else {
var oListItem = targetList.getItemById(selectedItems[i].id);
oListItem.set_item('Test', 'Rejected');
oListItem.update();
itemArray[i] = oListItem;
clientContext.load(itemArray[i]);
itemAllertYes.push(items[i].get_item('Title'));
clientContext.executeQueryAsync(onQueryFailed);
}
}
if (itemAllertNo != '') {
alert("List items " + itemAllertNo + " is rejected!");
}
if (itemAllertYes != '') {
alert("List items " + itemAllertYes + " successfully rejected!");
window.location.reload();
}
},
function (sender, args) {
alert('An error occured: ' + args.get_message());
});
}
function ApprovedItems() {
var context = SP.ClientContext.get_current();
var selectedItems = SP.ListOperation.Selection.getSelectedItems(context);
var ListGUID = SP.ListOperation.Selection.getSelectedList();
var clientContext = new SP.ClientContext.get_current();
var targetList = clientContext.get_web().get_lists().getById(ListGUID);
var itemArray = [];
var itemAllertNo = [];
var itemAllertYes = [];
getSelectedItems(function (items) {
for (var i = 0 ; i < items.length; i++) {
if ((items[i].get_item('Test') == "Aproved")) {
itemAllertNo.push(items[i].get_item('Title'));
} else {
var oListItem = targetList.getItemById(selectedItems[i].id);
oListItem.set_item('Test', 'Aproved');
oListItem.update();
itemArray[i] = oListItem;
clientContext.load(itemArray[i]);
itemAllertYes.push(items[i].get_item('Title'));
clientContext.executeQueryAsync(onQueryFailed);
}
}
if (itemAllertNo != '') {
alert("List items " + itemAllertNo + " is approved!");
}
if (itemAllertYes != '') {
alert("List items " + itemAllertYes + " successfully approved!");
window.location.reload();
}
},
function (sender, args) {
alert('An error occured: ' + args.get_message());
});
}
function onQueryFailed(sender, args) {
alert('Request failed. ' + args.get_message() + '\n' + args.get_stackTrace());
}
12. Open the Elements.xml in module ApprovedCA and remove default code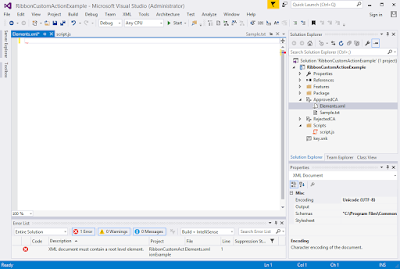
13. Add to code in this Elements.xml (ApprovedCA):
<?xml version="1.0" encoding="utf-8"?>
<Elements xmlns="http://schemas.microsoft.com/sharepoint/">
<CustomAction Id="f110dac1-9797-4959-8596-2161fb5a3cb1.RibbonCustomAction"
RegistrationType="List"
RegistrationId="100"
Location="CommandUI.Ribbon"
Sequence="10001"
Title="New Action Command">
<CommandUIExtension>
<CommandUIDefinitions>
<CommandUIDefinition Location="Ribbon.ListItem.Actions.Controls._children">
<Button Id="Approved Items"
Alt="Request RibbonCustomAction"
Sequence="100"
Command="New Action Command"
LabelText="Approved Items"
TemplateAlias="o1"
Image32by32="/_layouts/15/1033/images/formatmap32x32.png?rev=23" Image32by32Left="-375" Image32by32Top="-511"
Image16by16="/_layouts/15/1033/images/formatmap32x32.png?rev=23" Image16by16Left="-375" Image16by16Top="-511" />
</CommandUIDefinition>
</CommandUIDefinitions>
<CommandUIHandlers>
<CommandUIHandler Command="New Action Command"
CommandAction="javascript: ApprovedItems();"
EnabledScript="var EnableDisableItem = function()
{
this.clientContext = SP.ClientContext.get_current();
this.selectedItems = SP.ListOperation.Selection.getSelectedItems(this.clientContext);
if (selectedItems.length==1) {
if (selectedItems[0].fsObjType == 0)
{return true;}
else
{return false;}
}
if (selectedItems.length!=1)
{return false;}
};
EnableDisableItem();"/>
</CommandUIHandlers>
</CommandUIExtension >
</CustomAction>
<CustomAction
ScriptSrc="Scripts/script.js"
Location="ScriptLink"
Sequence="100">
</CustomAction>
</Elements>
14. Open the Elements.xml in module RejectedCA, remove default code and add to code in Elements.xml:
<?xml version="1.0" encoding="utf-8"?>
<Elements xmlns="http://schemas.microsoft.com/sharepoint/">
<CustomAction Id="f110dac1-9797-4959-8596-2161fb5a3cb2.RibbonCustomAction"
RegistrationType="List"
RegistrationId="100"
Location="CommandUI.Ribbon"
Sequence="10001"
Title="Invoke 'RibbonCustomAction' action">
<CommandUIExtension>
<CommandUIDefinitions>
<CommandUIDefinition Location="Ribbon.ListItem.Actions.Controls._children">
<Button Id="New Action Command"
Alt="Request RibbonCustomAction"
Sequence="100"
Command="Invoke_RibbonCustomActionButtonRequest"
LabelText="Rejected Items"
TemplateAlias="o1"
Image32by32="/_layouts/15/1033/images/formatmap32x32.png?rev=23" Image32by32Left="-375" Image32by32Top="-511"
Image16by16="/_layouts/15/1033/images/formatmap32x32.png?rev=23" Image16by16Left="-375" Image16by16Top="-511" />
</CommandUIDefinition>
</CommandUIDefinitions>
<CommandUIHandlers>
<CommandUIHandler Command="Invoke_RibbonCustomActionButtonRequest"
CommandAction="javascript: RejectedItems();"
EnabledScript="var EnableDisableItem = function()
{
this.clientContext = SP.ClientContext.get_current();
this.selectedItems = SP.ListOperation.Selection.getSelectedItems(this.clientContext);
if (selectedItems.length==1) {
if (selectedItems[0].fsObjType == 0)
{return true;}
else
{return false;}
}
if (selectedItems.length!=1)
{return false;}
};
EnableDisableItem(); "/>
</CommandUIHandlers>
</CommandUIExtension>
</CustomAction>
<CustomAction
ScriptSrc="Scripts/script.js"
Location="ScriptLink"
Sequence="100">
</CustomAction>
</Elements>
Happy Coding!
No comments:
Post a Comment